Top 100 full-stack developer interview questions and answers
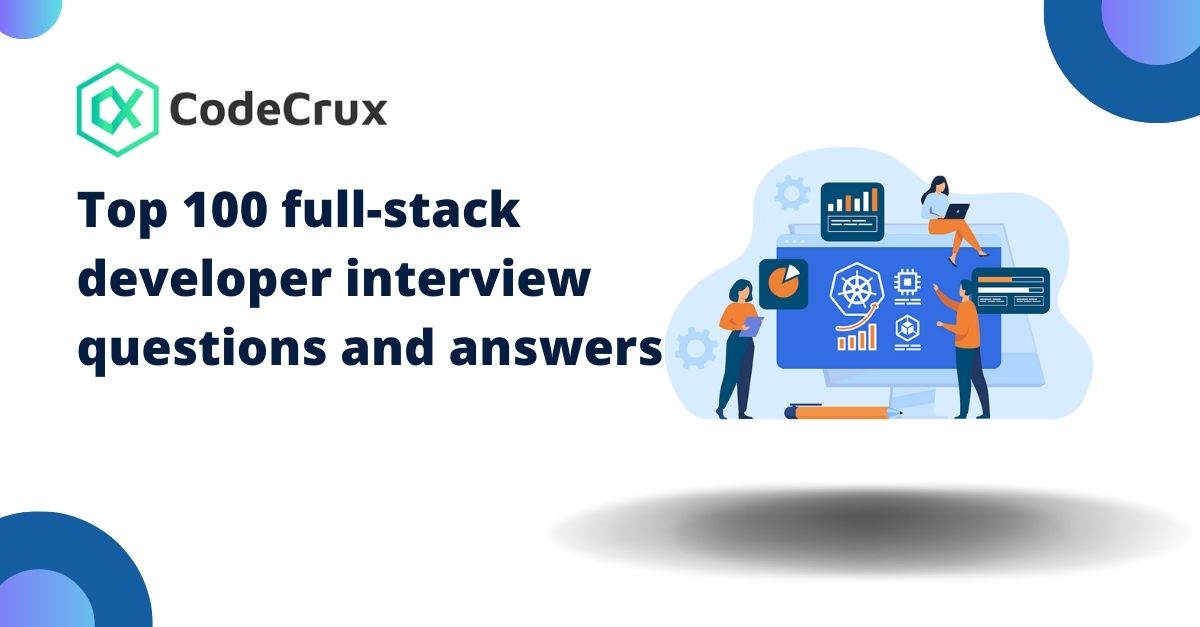
Here’s a comprehensive list of top 100 full-stack developer interview questions and answers covering front-end, back-end, database, and general topics. Due to the character limit, I’ll list key questions and answers in various categories. Let me know if you’d like this expanded or need a document for easier reference.
General Full-Stack Questions
-
What is a Full-Stack Developer?
A full-stack developer works on both front-end (client-side) and back-end (server-side) parts of a web application. - What is the difference between full-stack and MEAN/MERN stack?
- MEAN: MongoDB, Express.js, Angular, Node.js
- MERN: MongoDB, Express.js, React, Node.js
These are JavaScript-based stacks, while “full-stack” is a general term for working on both front-end and back-end.
-
What is RESTful API?
REST (Representational State Transfer) is an architectural style for designing networked applications using stateless communication via HTTP. - What is the difference between SQL and NoSQL databases?
- SQL: Relational, structured schema (e.g., MySQL, PostgreSQL).
- NoSQL: Non-relational, flexible schema (e.g., MongoDB, Cassandra).
- What are HTTP status codes?
- 200: OK
- 404: Not Found
- 500: Internal Server Error
Front-End Development
-
What is the difference between HTML and HTML5?
HTML5 introduced new tags like<article>
,<section>
,<audio>
,<video>
, and supports offline storage and multimedia. -
What is the DOM?
The DOM (Document Object Model) represents an HTML document as a tree structure where each node is an element. - What are CSS flexbox and grid?
- Flexbox: One-dimensional layout (row/column).
- Grid: Two-dimensional layout (rows and columns).
-
What are media queries in CSS?
Media queries allow you to apply styles based on device properties like screen width (@media screen and (max-width: 768px)
). - What is React Virtual DOM?
React’s Virtual DOM is a lightweight copy of the DOM, used for efficient UI updates by minimizing direct DOM manipulation.
JavaScript
- What is the difference between
var
,let
, andconst
?var
: Function-scoped, hoisted.let
: Block-scoped, not hoisted.const
: Block-scoped, cannot be reassigned.
-
What is asynchronous JavaScript?
Asynchronous JavaScript (e.g., using Promises orasync/await
) allows non-blocking operations like API calls. -
What is the purpose of closures?
Closures allow a function to access variables from its outer scope even after the outer function has returned. - What are JavaScript frameworks commonly used in full-stack development?
- Front-end: React, Angular, Vue.js.
- Back-end: Node.js, Express.js.
- What is CORS?
Cross-Origin Resource Sharing (CORS) allows restricted resources on a web page to be requested from another domain.
Back-End Development
-
What is Node.js?
Node.js is a JavaScript runtime built on Chrome’s V8 engine, enabling server-side scripting. -
What is middleware in Express.js?
Middleware functions handle requests, responses, or errors in Express.js applications. - What is the difference between monolithic and microservices architecture?
- Monolithic: Single codebase.
- Microservices: Decoupled, independently deployable services.
-
What is JWT?
JSON Web Token is used for securely transmitting information between parties as a JSON object, often for authentication. - What are some popular backend frameworks?
- Node.js (Express.js, Nest.js)
- Python (Django, Flask)
- Ruby (Ruby on Rails)
Database
-
What is indexing in databases?
Indexing improves database query performance by creating a data structure that allows quick data retrieval. - What is the difference between
DELETE
,DROP
, andTRUNCATE
in SQL?DELETE
: Removes specific rows.TRUNCATE
: Removes all rows, faster.DROP
: Deletes the table itself.
-
What is ACID in database transactions?
ACID stands for Atomicity, Consistency, Isolation, Durability, ensuring reliable transactions. -
What is MongoDB, and how does it store data?
MongoDB is a NoSQL database that stores data in JSON-like documents. - What are database relationships?
- One-to-One
- One-to-Many
- Many-to-Many
DevOps and Deployment
-
What is Docker?
Docker is a platform for containerizing applications, ensuring they run consistently in any environment. -
What is CI/CD?
Continuous Integration/Continuous Deployment automates code integration, testing, and deployment pipelines. -
What is the purpose of load balancing?
Distributes network traffic across multiple servers to ensure high availability and reliability. - What is the difference between a virtual machine and a container?
- VM: Full OS with hardware virtualization.
- Container: Lightweight, shares the host OS kernel.
- What is Kubernetes?
Kubernetes is an orchestration tool for deploying, scaling, and managing containerized applications.
Miscellaneous
-
What is the purpose of version control (e.g., Git)?
Version control manages changes to source code, allowing collaboration and history tracking. -
What are WebSockets?
WebSockets enable full-duplex communication between a client and server in real-time. -
What is GraphQL?
GraphQL is a query language and runtime for APIs, providing more flexibility than REST. -
What is CSRF and how to prevent it?
Cross-Site Request Forgery is prevented by using tokens, same-site cookies, or header validations. -
What is Webpack?
Webpack is a module bundler that bundles JavaScript files and assets for deployment.