Security Best Practices for Ruby on Rails Applications
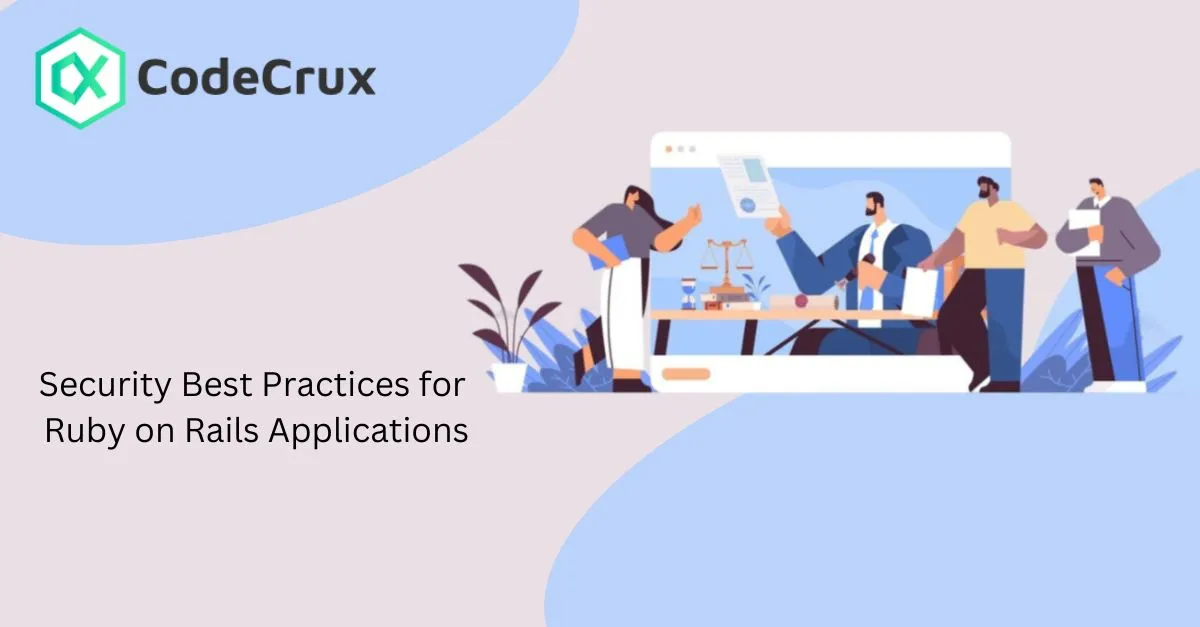
Ruby on Rails (RoR) is a robust and popular framework for building web applications. While it provides many built-in security features, developers must actively implement best practices to safeguard applications from evolving threats. In this blog, we’ll explore essential security measures for keeping your Rails applications secure.
1. Update Rails and Dependencies Regularly
Rails and its dependencies are frequently updated to patch security vulnerabilities. Ensure your application uses the latest stable version of Rails and its gems. Tools like bundler-audit
can help identify vulnerable dependencies.
Tips:
- Use
gem 'rails', '~> X.X'
to stay updated with minor and patch-level releases. - Automate dependency checking with tools like Dependabot or Snyk.
2. Enable Strong Parameters
Strong Parameters in Rails protect your application against mass assignment vulnerabilities by explicitly specifying which parameters are allowed.
Example:
ruby
def user_params
params.require(:user).permit(:name, :email, :password)
end
3. Sanitize User Input
User input can be a vector for attacks like SQL Injection and Cross-Site Scripting (XSS). Always sanitize and validate input data using Rails’ built-in helpers.
- Use
sanitize
orstrip_tags
for cleaning input. - Validate data at the model level.
Example:
ruby
validates :email, format: { with: URI::MailTo::EMAIL_REGEXP }
4. Use the Rails CSRF Protection
Rails automatically includes a CSRF (Cross-Site Request Forgery) token in forms and validates it on submission. Ensure it’s enabled by including protect_from_forgery
in your controllers.
Example:
ruby
class ApplicationController < ActionController::Base
protect_from_forgery with: :exception
end
5. Secure Sensitive Data with Rails Secrets
Store sensitive configuration data, such as API keys and database credentials, in Rails credentials or environment variables.
Steps:
- Use
rails credentials:edit
for secure storage. - Avoid committing sensitive data to your version control system.
6. Implement Authentication and Authorization
Authentication libraries like Devise simplify user authentication in Rails. For authorization, consider using gems like Pundit or CanCanCan to control user access.
Example:
ruby
class PostPolicy
def update?
user.admin? || user == record.user
end
end
7. Encrypt Data at Rest and in Transit
- Use HTTPS with SSL/TLS to encrypt data in transit.
- Store sensitive data like passwords with strong encryption algorithms.
Rails’ has_secure_password
is a convenient way to hash passwords:
ruby
class User < ApplicationRecord
has_secure_password
end
8. Enable HTTP Security Headers
Leverage middleware like SecureHeaders
to add security headers to your application. These headers prevent clickjacking, XSS, and other attacks.
Example:
ruby
config.middleware.use SecureHeaders::Middleware
SecureHeaders::Configuration.default do |config|
config.x_frame_options = "DENY"
config.x_content_type_options = "nosniff"
end
9. Perform Regular Security Audits
Use tools like Brakeman
to detect security vulnerabilities in your Rails application.
Install and run:
bash
gem install brakeman
brakeman
10. Restrict Public File Access
Limit access to files in your public
directory and ensure only necessary files are exposed.
11. Log and Monitor Security Events
Implement robust logging and monitoring solutions to detect and respond to suspicious activity. Gems like Lograge
can simplify log management in Rails.
12. Avoid Default Routes and Expose Only Needed Endpoints
Remove default routes and explicitly define routes in your routes.rb
file to avoid exposing unnecessary endpoints.
Example:
ruby
Rails.application.routes.draw do
resources :users, only: [:show, :update, :destroy]
end
13. Use a Web Application Firewall (WAF)
Enhance your application’s defense by using a WAF to block malicious traffic.
14. Protect Against DoS Attacks
Implement rate limiting to protect your application from denial-of-service (DoS) attacks. Gems like Rack::Attack
can help.
Example:
ruby
Rack::Attack.throttle('requests by ip', limit: 5, period: 2) do |req|
req.ip
end
Conclusion
Securing your Ruby on Rails application requires a combination of framework features, best practices, and proactive monitoring. By following these best practices, you can significantly reduce vulnerabilities and build a more secure application.
Remember, security is an ongoing process—stay informed about new threats and regularly update your skills and tools to keep your application safe.