Integrating AI and Machine Learning into React Native Apps
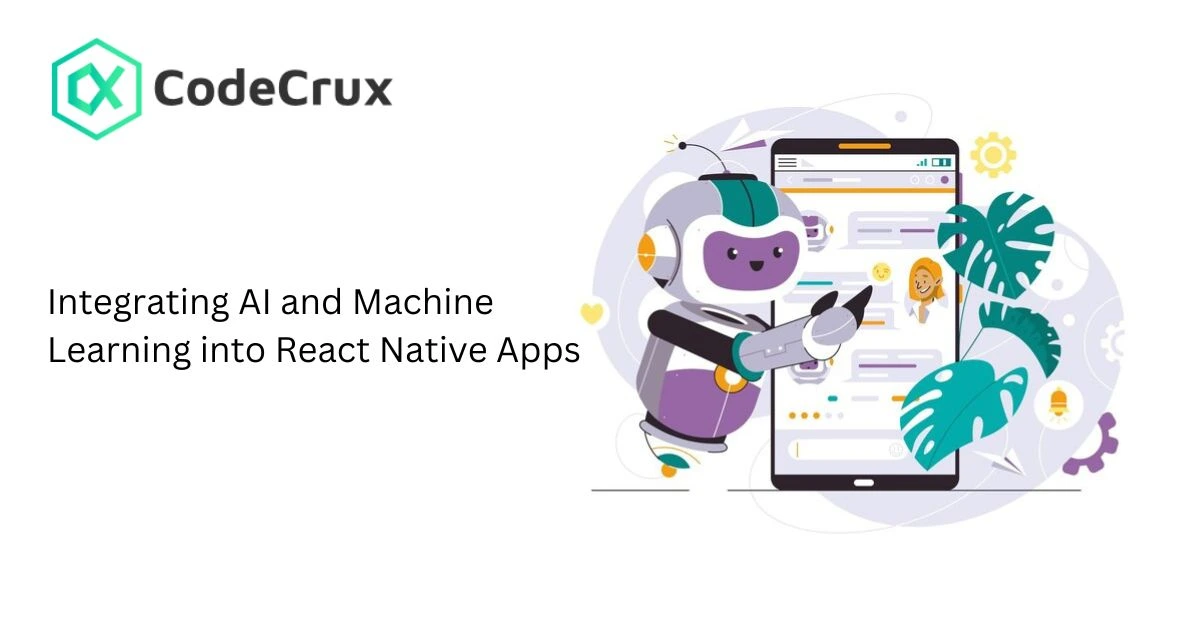
In the ever-evolving world of technology, Artificial Intelligence (AI) and Machine Learning (ML) are transforming industries, and mobile app development is no exception. When paired with React Native, a popular framework for building cross-platform mobile apps, AI/ML technologies can elevate your app’s functionality and user experience. This blog will guide you through the integration process, highlighting tools, libraries, and best practices.
Why Integrate AI/ML into React Native Apps?
AI and ML bring significant advantages to mobile applications, including:
- Personalization: Tailor experiences based on user behavior and preferences.
- Automation: Enable features like chatbots, voice recognition, and predictive analysis.
- Enhanced User Engagement: Improve interactions with features like image recognition and sentiment analysis.
- Competitive Edge: Differentiate your app with advanced capabilities.
Key Use Cases of AI/ML in React Native Apps
- Chatbots and Virtual Assistants: Integrate NLP-powered chatbots using libraries like
dialogflow
orrasa
. - Image and Video Analysis: Implement object detection or facial recognition with tools like
TensorFlow.js
. - Voice Commands: Enable voice-based navigation using libraries like
react-native-voice
. - Predictive Analytics: Analyze user patterns to provide proactive recommendations.
Steps to Integrate AI/ML into React Native Apps
1. Choose the Right ML Framework or Service
Popular frameworks and cloud services for AI/ML include:
- TensorFlow.js: For on-device ML capabilities.
- Google ML Kit: Pre-trained models for text recognition, face detection, and more.
- AWS Amplify with SageMaker: For integrating custom ML models.
- Microsoft Azure Cognitive Services: Offers pre-built AI APIs.
2. Set Up Your React Native Environment
Ensure your React Native project is properly configured with the latest version and necessary dependencies. Run:
bash
1
2
3
4
npx react-native init MyApp
cd MyApp
3. Install Required Libraries
Depending on your use case, install the necessary AI/ML libraries. For example:
-
To use TensorFlow.js:
bash
1 2
npm install @tensorflow/tfjs @tensorflow-models/mobilenet
-
To integrate Google ML Kit:
bash
1 2 3 4
npm install react-native-ml-kit
- Load and Use AI Models
Here’s a sample implementation of image recognition using TensorFlow.js:
javascript
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
import * as tf from '@tensorflow/tfjs';
import * as mobilenet from '@tensorflow-models/mobilenet';
import { useEffect } from 'react';
import { Image, View, Button } from 'react-native';
const App = () => {
useEffect(() => {
const loadModel = async () => {
const model = await mobilenet.load();
console.log('Model loaded successfully!');
};
loadModel();
}, []);
const classifyImage = async (imageUri) => {
const model = await mobilenet.load();
const predictions = await model.classify(imageUri);
console.log(predictions);
};
return (
<View>
<Image source= />
<Button title="Classify Image" onPress={() =>
classifyImage('image-uri-here')} />
</View>
);
};
export default App;
5. Test the Integration
Run your app using:
bash
1
2
3
4
npx react-native run-android
or
npx react-native run-ios
Ensure the AI features work seamlessly across both platforms.
Best Practices for AI/ML Integration
- Optimize for Performance: AI models can be resource-intensive. Use lightweight models or on-device processing when possible.
- Prioritize Privacy: Ensure user data is anonymized and stored securely.
- Test Thoroughly: AI features should be tested for accuracy and edge cases.
- Monitor and Iterate: Continuously monitor AI performance and update models to keep up with evolving data.
Challenges and How to Overcome Them
- Performance Bottlenecks: Use optimized models like TensorFlow Lite or on-device solutions to reduce latency.
- Cross-Platform Compatibility: Ensure libraries support both iOS and Android or use polyfills for unsupported functionalities.
- Model Training Complexity: Leverage pre-trained models or cloud-based services for easier integration.
Conclusion
Integrating AI and ML into React Native apps opens the door to innovative features and superior user experiences. By leveraging powerful libraries and adhering to best practices, you can create applications that are not only intelligent but also efficient and user-centric.
Whether you’re building a chatbot, implementing image recognition, or enabling voice commands, the synergy of React Native and AI/ML is a game-changer. Start small, iterate, and watch your app transform into a smarter, more dynamic product.